Learn how to build custom JavaScript grids from scratch with our 9-step guide. Become a pro in 2024 with our comprehensive tutorial. Start coding today!
JavaScript grids are a part of many web apps today. They allow us to present large, complex datasets in a tabular, structured format. And they also make your UI (user interface) look good, enhancing the user experience. However, creating JavaScript grids can be challenging. Moreover, adding advanced functionality like sorting, filtering, grouping, and pagination can be time-consuming. Fortunately, we have JavaScript frameworks that make it easier to create high-performance JS grids to handle large datasets efficiently. One such JavaScript framework is Ext JS.
Read on if you’re looking to create a custom JS grid for your web app. We'll show you how to create a custom and interactive JS grid using the Ext JS framework. Ext JS is a leading JavaScript framework. It has one of the fastest and most efficient JS data grids. The grid comes with lots of basic and advanced features. Another reason why we chose EXT JS is that creating a custom JavaScript grid with the framework is super easy. Let's get started!
Understanding the Basics
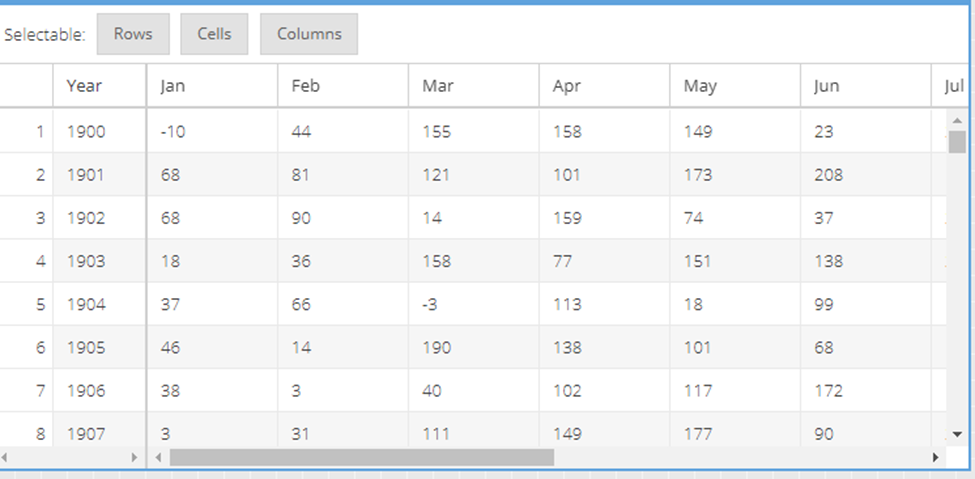
A JavaScript grid allows us to arrange and present data in tabular format in our web apps. It’s like a UI component that enables users to view, understand, and interact with data. JS grids are especially helpful for large datasets.
Core elements of a JavaScript grid include:
- Rows: Rows enable us to display the horizontal arrangements of data records in the grid. Rows are made up of cells, where each cell contains a single record/data value.
- Columns: Columns allow us to define the vertical structure of the grid. They represent specific aspects of the data, such as name, age, date, month, email ID, etc. One column contains various cells across different rows.
- Cells: A cell is a single element/unit in a grid. Each cell contains an individual data value specific to a row and column.
Choosing a Javascript Framework
When choosing a JS library or framework for creating a JS grid, it's essential to consider the following factors:
- Assess the ease of integration of the framework into your web application.
- Check whether the frameworks offer specific data grid features for your specific project, such as sorting and filteringmization options. Choose a framework that provides flexibility in customising the grid elements and appearance of the grid.
- Choose a framework that has extensive documentation and an active developer community. This will make it easier to solve any issues you may encounter while integrating the JavaScript framework and implementing the grid.
While there are numerous JavaScript frameworks, we've selected the Ext JS framework for this guide for various reasons. Ext JS is one of the leading modern javascript frameworks. It offers over 140 high-performance, customisable, and thoroughly tested UI components, including a blazing-fast JS data grid.
You’ll find all sorts of basic and advanced grid features in the Ext JS data grid. These include grouping, locking, sorting, filtering, pagination, and row expansion. The grid can handle thousands of records efficiently without affecting performance. Moreover, Ext JS integrates seamlessly with any modern web app and is cross-browser and cross-platform compatible. The framework also has extensive documentation and a great community.
Setting Up Your Development Environment
Install Node.js and npm
The first step to creating a JS grid with a framework is installing the framework and setting up your development environment. To do this, first download Node.js and npm.
You can download the Ext JS zip file from Sencha's official website. You can install Sencha Cmd, which is a command-line tool for building user interfaces for Sencha apps. Or you can install packages directly from npm:
$ npm install -g @sencha/ext-gen
|
Choosing a Code Editor
Next, we need a code editor. Select an easy-to-use code editor and look for features like code completion, syntax highlighting, debugging support, etc. Popular options include Visual Studio Code, UltraEdit, and Sublime Text.
Setting Up Version Control with Git
Git is essentially a version control system. It allows developers to:
- Manage codebase efficiently
- Track changes
- Collaborate with other developers
- Deploy the app
To set up version control with Git, first download and install Git. Then, configure Git (by setting up your username and email ID), create a new repository (git init) and commit changes.
Designing the Grid Structure
First, we have to define the grid requirements and specifications, such as:
- The type of data we want to display on the grid, such as static data or dynamically fetched
- The fields each item in the grid will contain
- Features required in the grid, such as grouping, pagination, sorting, editing, filtering, etc.
Once you've defined your requirements, sketch a grid layout using a tool like Figma. Key elements of the grid layout include:
- Column titles
- The arrangement of rows and columns
- The height and width of rows and columns
- Headers
- Additional components like buttons or toolbars.
Implementing HTML Structure
Below is the code for the HTML file, which includes the necessary Ext JS libraries.
<head>
<title>Custom JavaScript Grid with Ext JStitle>
<link rel="stylesheet" href="https://cdn.sencha.com/ext/gpl/7.5.0/build/packages/ext-theme-neptune/build/resources/ext-theme-neptune-all.css">
<script src="https://cdn.sencha.com/ext/gpl/7. 5.0/build/ext-all.js">script>
<script src="app.js">script>
head>
<body>
<div id="grid-container">div>
body>
html>
|
We've created a container div with the id grid-container in the above code. This is where our Ext JS grid will be rendered.
Now, we'll create a JavaScript file named app.js. This file will contain the code to create the grid. Here is an example code for creating the initial structure for rows and columns in an Ext JS grid and binding the grid component to a data store in an Ext JS app:
Ext.onReady(function () {
// Define your data model
Ext.define('MyModel', {
extend: 'Ext.data.Model',
fields: [
{ name: 'id', type: 'int' },
{ name: 'name', type: 'string' },
// Add more fields as needed
]
});
// Create a data store
var store = Ext.create('Ext.data.Store', {
model: 'MyModel',
data: [
{ id: 1, name: 'Row 1' },
{ id: 2, name: 'Row 2' },
// Add more data as needed
]
});
// Create the grid
var grid = Ext.create('Ext.grid.Panel', {
renderTo: 'grid-container', // Render grid to the specified container
store: store,
columns: [
{ text: 'ID', dataIndex: 'id' },
{ text: 'Name', dataIndex: 'name' },
// Add more columns as needed
],
height: 300,
width: 400,
title: 'Custom Grid'
});
});
|
In the above code, we've:
- Defined a data model (MyModel), which basically represents the grid data structure.
- Created a data store using our defined model and defined sample data.
- Finally, we created the grid panel using Ext.grid.Panel and defined columns to display data from the store.
Styling with CSS
Now, we'll customize the grid's appearance to ensure it looks attractive. Here, you can also define grid adjustment for smaller screens for responsive design. Here is an example CSS code for styling the grid:
/* Basic styling for grid container */
#grid-container {
margin: 20px;
}
@media only screen and (max-width: 600px) {
/* Adjust grid width for smaller screens */
#grid-container {
width: 100%;
}
}
/* Custom styles for rows and columns */
.x-grid-row {
background-color: #f9f9f9; /* Light gray background for rows */
}
.x-grid-cell {
padding: 5px; /* Add padding to cells */
border-bottom: 1px solid #ddd; /* Add bottom border to cells */
}
|
In the above code, we've:
- Applied basic styling to the grid container with some margin.
- Adjusted the grid width for smaller screens to make our grid responsive. You can also utilize Ext JS "Ext.layout.container.Responsive" layout for responsive design. This layout allows you to define how the components should adapt based on screen size.
- Applied custom styles for rows and columns, such as light grey background, padding, and bottom border for cells.
You can customize the grid further according to your design requirements.
Adding Interactivity with JavaScript
We basically use event handlers and event listeners to handle user interactions and interactivity in JavaScript.
Ext.onReady(function () {
// Define your data model
Ext.define('MyModel', {
extend: 'Ext.data.Model',
fields: [
{ name: 'id', type: 'int' },
{ name: 'name', type: 'string' },
// Add more fields as needed
]
});
// Create a data store
var store = Ext.create('Ext.data.Store', {
model: 'MyModel',
data: [
{ id: 1, name: 'Row 1' },
{ id: 2, name: 'Row 2' },
// Add more data as needed
]
});
// Create the grid
var grid = Ext.create('Ext.grid.Panel', {
renderTo: 'grid-container', // Render grid to the specified container
store: store,
columns: [
{ text: 'ID', dataIndex: 'id' },
{ text: 'Name', dataIndex: 'name' },
// Add more columns as needed
],
height: 300,
width: 400,
title: 'Custom Grid',
listeners: {
// Event listener for row click
itemclick: function(view, record, item, index, e, eOpts) {
console.log('Row clicked:', record.get('name'));
// Retrieve data from the clicked row
var clickedId = record.get('id');
var clickedName = record.get('name');
// Perform actions based on the clicked row data
alert('Clicked row ID: ' + clickedId + ', Name: ' + clickedName);
},
// Event listener for row hover
itemmouseenter: function(view, record, item, index, e, eOpts) {
console.log('Row hovered:', record.get('name'));
// Add logic here to handle row hover event
// For example, you can highlight the hovered row or display additional information
item.style.backgroundColor = '#f0f0f0'; // Change background color
},
// Event listener for mouse leave row
itemmouseleave: function(view, record, item, index, e, eOpts) {
console.log('Row mouse leave:', record.get('name'));
// Restore original background color when mouse leaves row
item.style.backgroundColor = ''; // Restore original background color
}
}
});
});
|
Implementing Advanced Features
Depending on your dataset and requirements, you can add more features to your grid. Ext JS grid supports several advanced grid features. These include sorting, filtering, grouping, locking, and more.
Here is an example code for adding filtering to your grid:
// Add event listener for filtering
store.on('filterchange', function() {
console.log('Filter changed:', store.getFilters().items);
// Custom logic to handle filter change
// For example, you can update UI elements or perform additional actions based on filter changes
var appliedFilters = store.getFilters().items;
// Add logic here to react to filter changes
|
Testing and Debugging
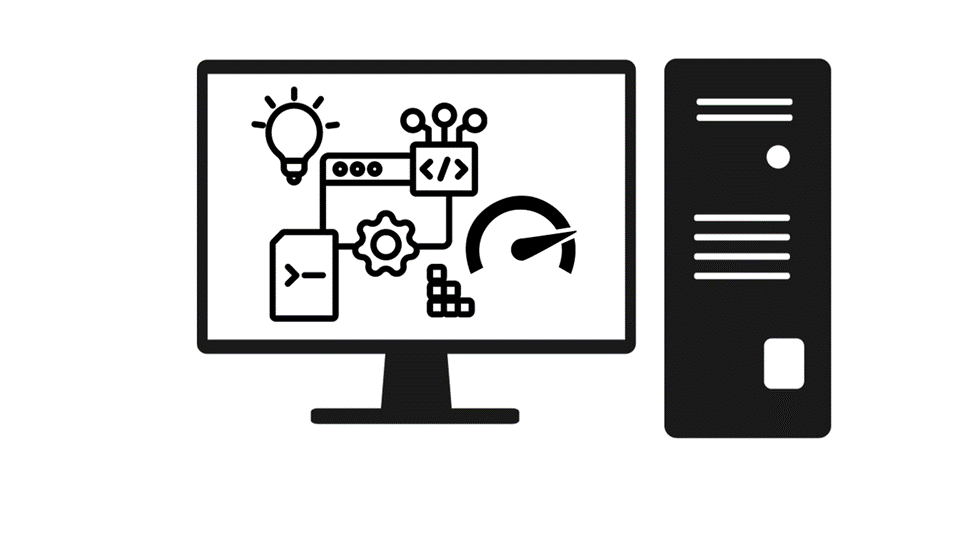
Testing and debugging web components are essential for creating flawless UIs that work seamlessly on any browser. Similarly, testing your grid components is crucial. Here are some tips for testing your grid components
- Write unit tests for your grid components. You can use tools and frameworks like Jasmine and Mocha.
- Make sure to write tests for the behaviour of grid components. This includes filtering, sorting, pagination, and data manipulation.
- Leverage browser developer tools like Chrome DevTools to inspect grid elements and debug layout issues.
- Use console.log() statements in your JS code to get the output debug information and inspect the values of variables during runtime.
- Use cross-browser testing tools like Sauce Labs and BrowserStack to effectively test your grid components on multiple browsers.
Elevate your web projects with Sencha's cutting-edge framework – Get started!
Optimization and Performance Tuning
Identify and assess performance bottlenecks. These can include memory usage and network requests. Finally, implement optimizations for faster rendering.
Conclusion
A JavaScript data grid lets us present large datasets in our web app in a structured, tabular format. However, creating custom JS grids can be challenging and time-consuming, especially if you’re making them from scratch. Fortunately, we can use a JavaScript framework like Ext JS to build high-performance JS grids quickly and efficiently. Here are the key steps to follow while creating a JavaScript grid:
- Understand the essential aspects of a JavaScript grid, such as rows, columns, and cells.
- Set up your development environment. This includes installing Node.js and npm, choosing a code editor, and setting up version control with Git.
- Define the requirements and specifications of the grid and sketch the grid layout.
- Create an HTML file, set up the grid container and add the initial structure for rows and columns.
- Style your grid with CSS.
- Add interactivity to your grid with event listeners
- Add advanced features like filtering, sorting, and pagination to your grid based on your requirements.
- Test and debug your grid component.
FAQ:
Do I need prior experience in JavaScript to follow this guide?
A basic understanding of HTML, CSS, and JavaScript core concepts, such as event listener, will make it easier to follow this guide.
Why should I invest time in building custom JavaScript grids?
Building custom JavaScipt allows you to style your grids to match your web app's overall design. Moreover, with custom grids, you can define rows and columns depending on your specific dataset and add additional features like sorting, filtering, and grouping.
How do I troubleshoot if I encounter issues during the testing and debugging phase?
You can check the console, review your code, and use debugging tools.